7. Two Ways to set up a firebase with an application(Using Firebase CLI (5.1) OR Manually(5.2)).
7.1. Setting up Firebase for a Flutter project using the Firebase CLI involves several steps. Below is a detailed guide on how to do it:
7.1.1. Install Firebase CLI:
First, you need to install the Firebase CLI if you haven’t already. You can install it via npm (Node Package Manager) by running the following command in your terminal or command prompt:

7.1.2. Login to Firebase:
After installing the Firebase CLI, log in to your Firebase account by running the following command:

This will open a browser window prompting you to log in to your Google account associated with Firebase.
7.1.3. Create a Firebase Project:
If you haven’t already created a Firebase project, you can create one using the Firebase CLI by running:

Follow the prompts to create a new project.
7.1.4. Initialize Firebase in Your Flutter Project:
Navigate to your Flutter project directory in the terminal.
Initialize Firebase in your project by running:

This command will prompt you to select the Firebase features you want to set up. Choose the Firebase services you intend to use, such as Authentication, Firestore, etc.
7.1.5. Configure Firebase:
During the initialization process (firebase init)
, you’ll be asked to select the Firebase project you created or configured earlier.
Choose the appropriate project from the list.
7.1.6. Install Required Dependencies:
After selecting the Firebase features, Firebase CLI will generate the necessary configuration files for your project.
Next, you need to install the required Firebase packages in your Flutter project. You can do this by adding the dependencies to your pubspec.yaml
file:
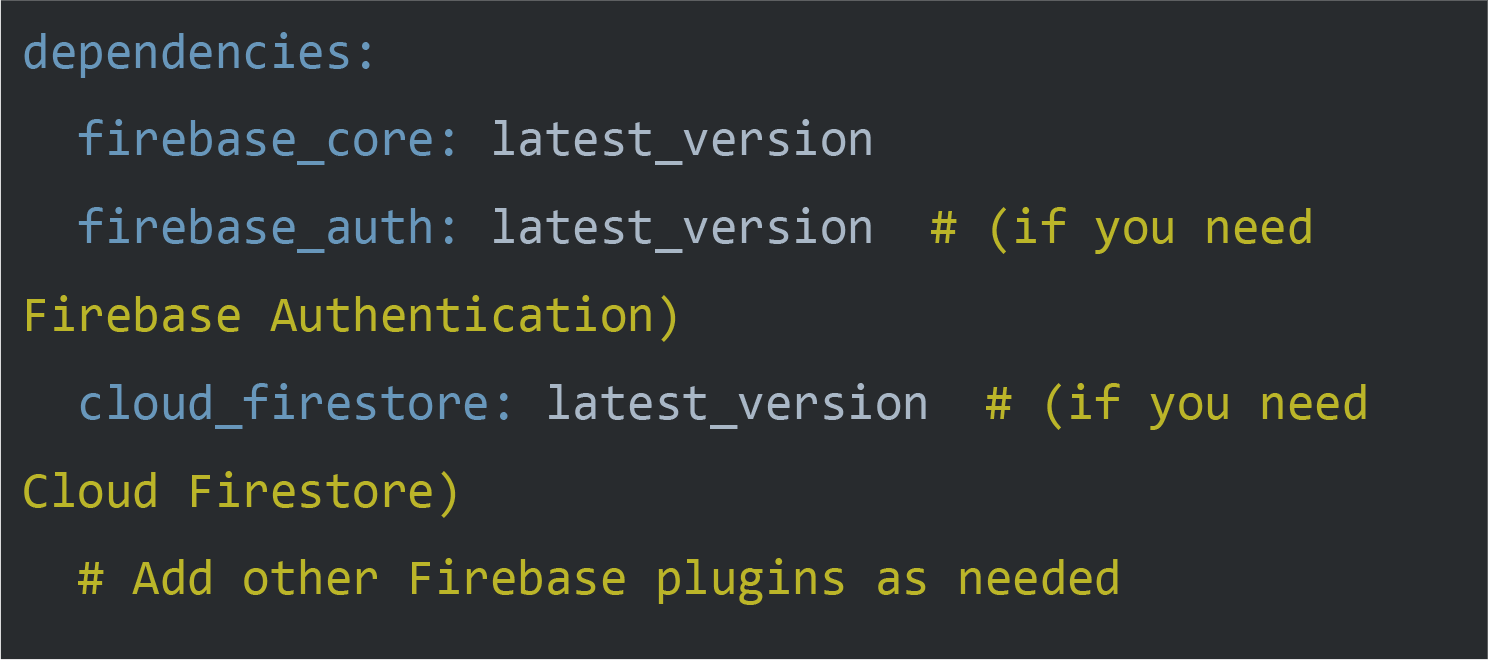
Replace latest_version
with the actual version numbers of the Firebase plugins you want to use. You can find the latest versions on pub.dev.
7.1.7. Initialize Firebase in Your Flutter App:
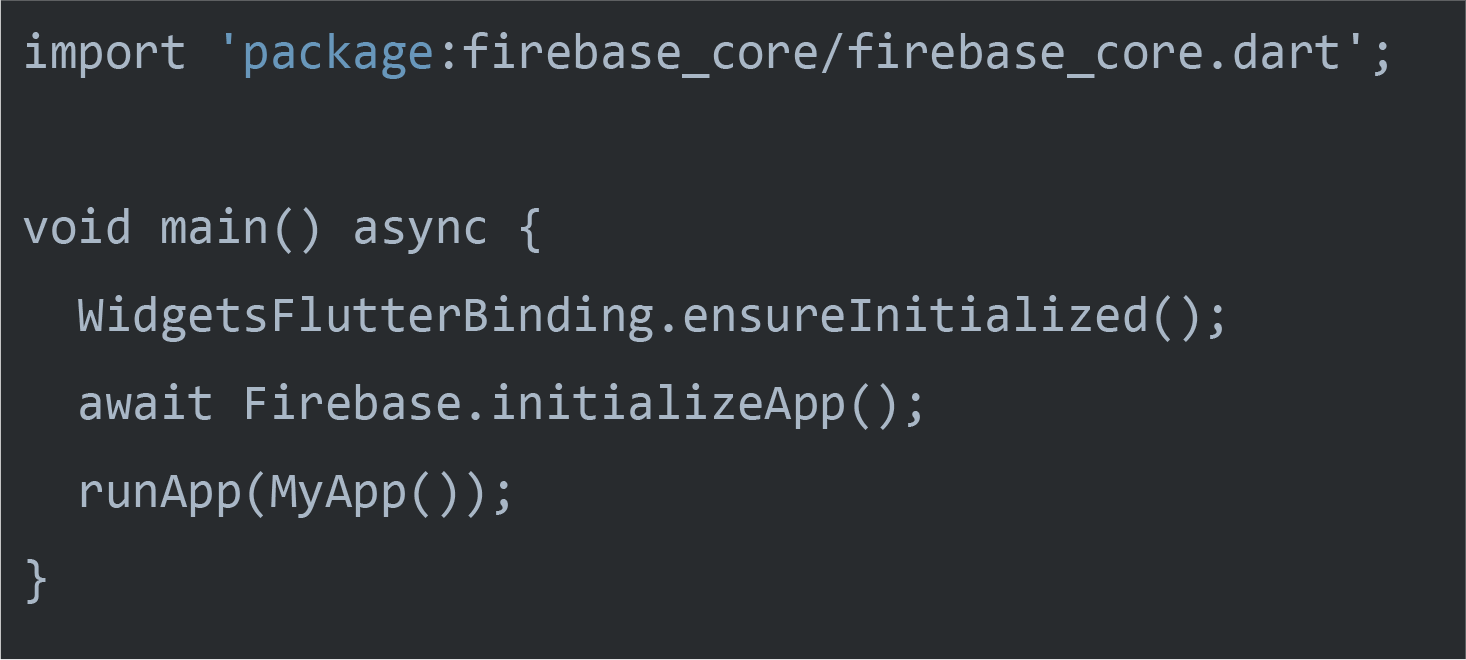
7.1.8. Using Firebase Services:
Now you can use Firebase services in your Flutter app. Import the necessary Firebase packages and follow the respective documentation for each service you want to use (e.g., Firebase Authentication, Cloud Firestore).
7.1.9. Testing:
Run your Flutter app on a device or emulator to verify that Firebase services are correctly integrated and working.
7.2. Configuring and setting up FlutterFire in your Flutter project involves several steps. Here’s a detailed guide on how to do it:
7.2.1. Create a Firebase Project:
Go to the Firebase Console: https://console.firebase.google.com/
Click on “Add Project” and follow the instructions to create a new project.
Once your project is created, you’ll be redirected to the project dashboard.
7.2.2. Add an App to Your Firebase Project:
In the Firebase console, select your project.
Click on the “Add app” button (usually represented by an Android or iOS icon).
Follow the setup instructions to register your app with Firebase.
Download the google-services.json
file for Android or GoogleService-Info.plist
file for iOS. These files contain your Firebase project configuration details.
7.2.3. Flutter Project Setup:
Open your Flutter project in your preferred editor.
Navigate to the android/app
directory for Android or ios/Runner
directory for iOS, and paste the google-services.json
(for Android) or GoogleService-Info.plist
(for iOS) file that you downloaded earlier.
7.2.4. Add Firebase SDK to Your Flutter Project:
Open your Flutter project’s pubspec.yaml
file.
Add the following dependencies:
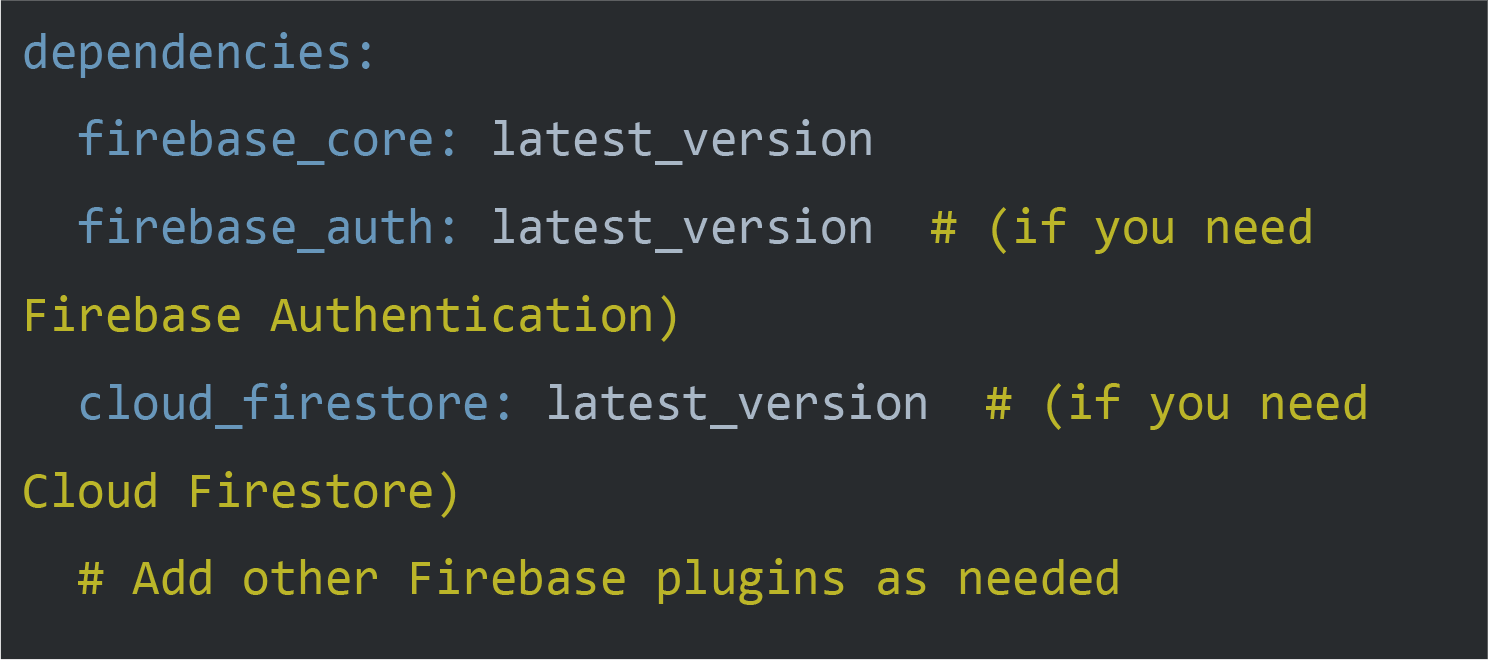
Replace latest_version
with the latest version numbers of the Firebase plugins you want to use. You can find the latest versions on pub.dev.
7.2.5. Using Firebase Services:
You can now use Firebase services in your Flutter app. Import the necessary Firebase packages and follow the respective documentation for each service you want to use (e.g., Firebase Authentication, Cloud Firestore).
7.2.6. Testing:
Run your Flutter app on a device or emulator to verify that Firebase services are correctly integrated and working.